前言
本文基于4.27
本文记录最近工作中遇到的与材质系统相关的内容
UMaterial
1
| class UMaterial : public UMaterialInterface
|
图上这些是材质的输入节点,类型有FScalarMaterialInput、FColorMaterialInput、FVectorMaterialInput等,都继承自FMaterialInput。
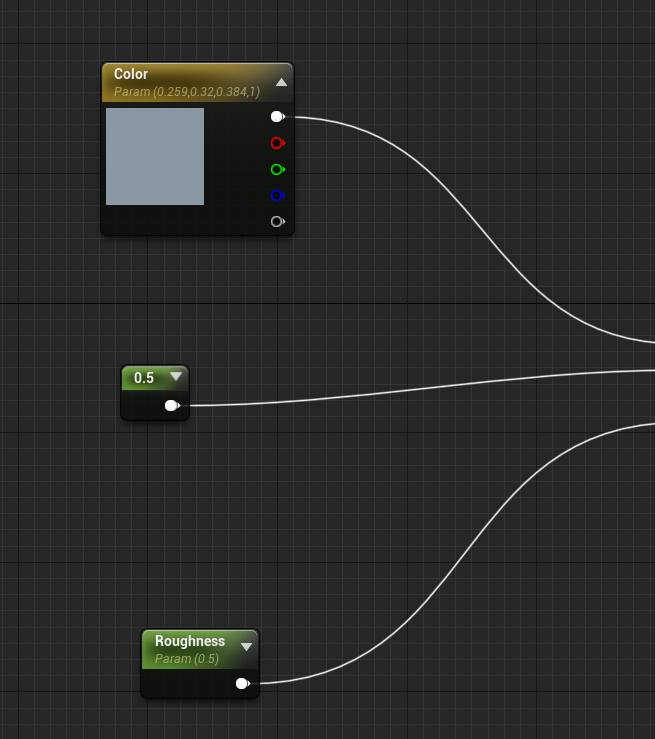
这些是材质表达式。
获取输入到材质的某一个MaterialProperty的表达式
方法:
1
| FExpressionInput* GetExpressionInputForProperty(EMaterialProperty InProperty);
|
参数:
枚举类型,表示材质的某一个属性,比如BaseColor、Metallic、Roughness等,定义在SceneTypes.h中
返回值:
返回一个ExpressionInput的指针,表示的是输入到这个材质属性的一个表达式。如果没有的话会返回一个空指针。
比如前面的图里的Engine自带的材质M_Basic_Floor来讲,其中BaseColor的输入是Color,那么通过如下调用:
1
| FExpressionInput* BaseColorInput = Material->GetExpressionInputForProperty(EMaterialProperty::MP_BaseColor);
|
就可以得到BaseColor的ExpressionInput,在通过类型转换可以确认具体的表达式类型并进行进一步的操作。
获取材质实例的某种类型的参数
方法:
1 2 3 4 5 6 7 8 9 10 11 12 13
| void GetAllParametersOfType(EMaterialParameterType Type, TArray<FMaterialParameterInfo>& OutParameterInfo, TArray<FGuid>& OutParameterIds) const;
ENGINE_API virtual void GetAllScalarParameterInfo(TArray<FMaterialParameterInfo>& OutParameterInfo, TArray<FGuid>& OutParameterIds) const override; ENGINE_API virtual void GetAllVectorParameterInfo(TArray<FMaterialParameterInfo>& OutParameterInfo, TArray<FGuid>& OutParameterIds) const override; ENGINE_API virtual void GetAllTextureParameterInfo(TArray<FMaterialParameterInfo>& OutParameterInfo, TArray<FGuid>& OutParameterIds) const override; ENGINE_API virtual void GetAllRuntimeVirtualTextureParameterInfo(TArray<FMaterialParameterInfo>& OutParameterInfo, TArray<FGuid>& OutParameterIds) const override; ENGINE_API virtual void GetAllFontParameterInfo(TArray<FMaterialParameterInfo>& OutParameterInfo, TArray<FGuid>& OutParameterIds) const override;
#if WITH_EDITORONLY_DATA ENGINE_API virtual void GetAllMaterialLayersParameterInfo(TArray<FMaterialParameterInfo>& OutParameterInfo, TArray<FGuid>& OutParameterIds) const override; ENGINE_API virtual void GetAllStaticSwitchParameterInfo(TArray<FMaterialParameterInfo>& OutParameterInfo, TArray<FGuid>& OutParameterIds) const override; ENGINE_API virtual void GetAllStaticComponentMaskParameterInfo(TArray<FMaterialParameterInfo>& OutParameterInfo, TArray<FGuid>& OutParameterIds) const override; #endif
|
使用方式,用GetAllScalarParameterInfo举例
1 2 3 4 5 6 7 8 9 10 11 12
| TArray<FMaterialParameterInfo> ScalarParameterInfos; TArray<FGuid> ScalarParameterIds; MaterialInstance->GetAllScalarParameterInfo(ScalarParameterInfos, ScalarParameterIds);
for (FMaterialParameterInfo ParameterInfo : ScalarParameterInfos) { FString ParameterName = ParameterInfo.Name.ToString(); float ParameterValue; MaterialInstance->GetScalarParameterValue(ParameterInfo, ParameterValue);
}
|
实际上GetAllScalarParameterInfo内部调用的是GetAllParametersOfType,然后第一个参数传了EMaterialParameterType::Scalar
1 2 3 4
| void UMaterialInstance::GetAllScalarParameterInfo(TArray<FMaterialParameterInfo>& OutParameterInfo, TArray<FGuid>& OutParameterIds) const { GetAllParametersOfType(EMaterialParameterType::Scalar, OutParameterInfo, OutParameterIds); }
|
获取StaticSwitchParameter节点的输入
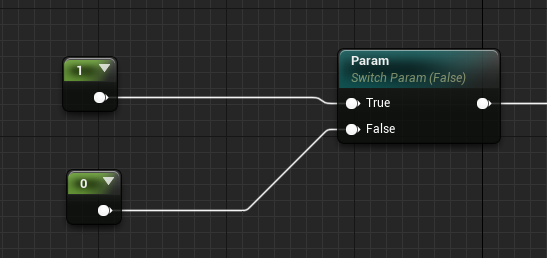
1 2 3 4 5 6 7
| TArray<UMaterialExpressionStaticSwitchParameter*> MaterialExpressionStaticSwitchParameters; Material->GetAllExpressionsInMaterialAndFunctionsOfType(MaterialExpressionStaticSwitchParameters); for (auto& ExpressionStaticSwitchParameter : MaterialExpressionStaticSwitchParameters) { FExpressionInput* SwitchTrueInput = ExpressionStaticSwitchParameter->GetInput(0); FExpressionInput* SwitchFalseInput = ExpressionStaticSwitchParameter->GetInput(1); }
|